What is an Ultrasonic Sensor?
The HC-SR04 ultrasonic sensor measures distances by emitting high-frequency sound pulses that bounce off objects and return to the sensor.
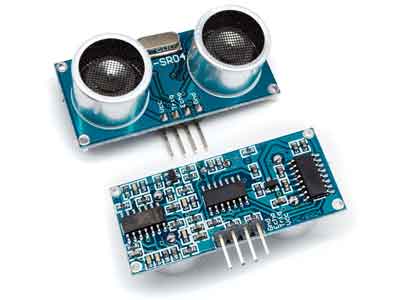
How Does it Work?
By measuring the time between the emission and return of a sound pulse, the sensor calculates distance. The speed of sound (343 m/s) is used for these calculations.
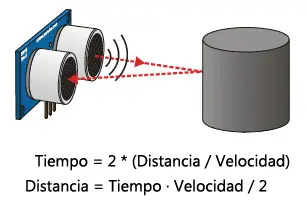
Electrical Schematic
The circuit schematic for connecting the HC-SR04 is shown below:
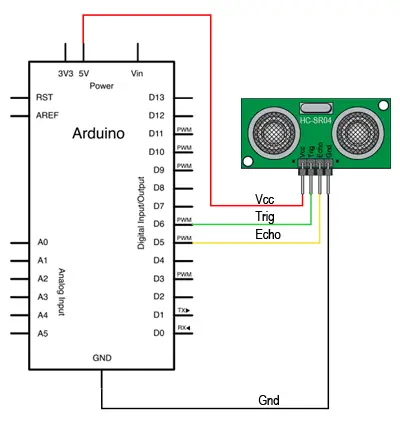
Assembly Diagram
The assembly on a breadboard is illustrated as follows:
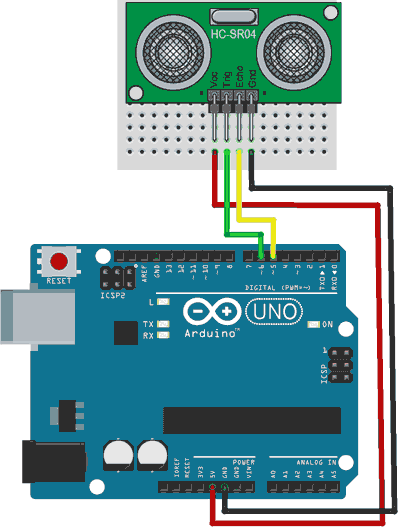
Code Example (Without Library)
Below is an example code to measure distance using the HC-SR04 sensor without any external library:
const int EchoPin = 5;
const int TriggerPin = 6;
void setup() {
Serial.begin(9600);
pinMode(TriggerPin, OUTPUT);
pinMode(EchoPin, INPUT);
}
void loop() {
int cm = ping(TriggerPin, EchoPin);
Serial.print("Distance: ");
Serial.println(cm);
delay(1000);
}
int ping(int TriggerPin, int EchoPin) {
long duration, distanceCm;
digitalWrite(TriggerPin, LOW); // Clean pulse
delayMicroseconds(4);
digitalWrite(TriggerPin, HIGH); // Trigger pulse
delayMicroseconds(10);
digitalWrite(TriggerPin, LOW);
duration = pulseIn(EchoPin, HIGH); // Measure duration
distanceCm = duration * 10 / 292 / 2; // Convert to cm
return distanceCm;
}
Code Example (Using NewPing Library)
Using the NewPing library simplifies the process and provides additional features such as noise filtering:
#include
const int UltrasonicPin = 5;
const int MaxDistance = 200;
NewPing sonar(UltrasonicPin, UltrasonicPin, MaxDistance);
void setup() {
Serial.begin(9600);
}
void loop() {
delay(50); // Wait 50ms between pings
Serial.print(sonar.ping_cm()); // Get distance in cm
Serial.println(" cm");
}
Important Notes
The HC-SR04 sensor is sensitive to reflections and echoes. It is not suitable for outdoor environments or highly reflective surfaces.